Understanding Apache Flink Performance Challenges
Apache Flink is a strong tool for stream processing. But, it faces many performance hurdles. These include bad parallelism settings, poor state management, and wrong resource use. It’s key to tackle these to boost your Flink app’s speed.
Common Performance Bottlenecks
Finding and fixing common performance problems in Apache Flink is a big challenge. These issues can slow down your system. They include:
- Network Constraints: Too little bandwidth or slow data transfer can really slow things down.
- Serialization Issues: Bad serialization and deserialization can waste CPU time.
- Backpressure: When data comes in faster than it can be processed, backpressure happens. This makes things even slower.
Identifying Symptoms of Poor Performance
Spotting signs of poor performance in Flink apps is crucial. Finding these issues early can stop bigger problems. Common signs are:
- Longer times to process data.
- Often running out of memory.
- Slow task execution.
- Not using system resources well.
Knowing these signs and their causes helps developers fix Apache Flink performance issues. By addressing these, you can make your stream processing pipeline faster and more reliable.
Setting Up a Scalable Flink Cluster
Setting up a scalable Flink cluster is key to better Apache Flink app performance. It’s especially important for handling changing workloads. Choosing the right hardware and cloud options can make your cluster more scalable and stable.
When setting up a scalable Flink cluster, you need to think about your hardware needs. Important things to look at are:
- CPU: Make sure it can handle tough data processing tasks.
- Memory: Enough RAM helps jobs run smoothly and manage state well.
- Storage: Fast SSDs improve disk I/O, which is important for state backends and checkpoints.
- Networking: Good bandwidth and low-latency networks help data move quickly in the cluster.
Using cloud services can make setting up and scaling easier. Clouds like AWS, Google Cloud, and Azure offer many benefits:
- Elasticity: Easily scale your cluster up or down as needed.
- Managed Services: Let cloud providers handle maintenance for you.
- Cost Efficiency: Only pay for what you use.
- Autoscaling: Adjust resources automatically based on workload.
- Resource Management: Use Flink’s resource management, like Mesos or Kubernetes, to manage resources well.
- Monitoring and Alerts: Use strong monitoring tools to catch performance issues fast and set up alerts for important metrics.
- Regular Maintenance: Plan regular maintenance times for updates and optimizations without stopping workflows.
With careful planning and following these tips, your scalable Flink cluster will always improve Apache Flink app performance. This is true no matter the workload’s complexity or size.
Tuning Task Manager Configuration
To make Apache Flink run better, you need to tweak the task manager settings. This includes adjusting memory and parallelism levels. These changes can make your Flink apps work more efficiently. Let’s look at how to set up memory and parallelism in a Flink Task Manager.
Memory Configuration
Good memory management is key for smooth task manager operation. It prevents garbage collection pauses and out-of-memory errors. When tuning Apache Flink task managers, remember to consider JVM heap, metaspace, and off-heap memory.
- JVM Heap Memory: Allocate enough for user code and Flink’s needs. Adjust `taskmanager.memory.task.heap.size` based on your app’s needs.
- JVM Metaspace: Make sure you have enough for class metadata. Use `taskmanager.memory.jvm-metaspace.size` to set this.
- Off-Heap Memory: For network buffers and RocksDB, use `taskmanager.memory.managed.size` and `taskmanager.memory.network.fraction.
Getting these memory settings right makes your task processing better.
Parallelism Settings
Setting the right task parallelism is crucial. It helps spread tasks evenly across the cluster. This boosts throughput and resource use. Key points to think about include:
- Default Parallelism: Set with `parallelism.default. It’s the base parallelism for all operators. Pick a value that fits your cluster and workload.
- Max Parallelism: Use `parallelism.max` to set the top limit for parallelism. This stops your cluster from getting too busy.
- Operator-Specific Parallelism: For finer control, adjust parallelism for each operator in your Flink job code with `setParallelism(int parallelism).
Parameter | Description | Example Values |
---|---|---|
parallelism.default | Default parallelism for all operators | 4, 8, 16 |
parallelism.max | Maximum parallelism allowed | 128, 256 |
taskmanager.memory.task.heap.size | Heap size allocation for task slots | 1g, 2g |
taskmanager.memory.managed.size | Managed memory size | 512m, 1g |
By carefully adjusting these settings, your Flink Task Manager will meet your workload’s needs better.
Optimizing Job Parallelism
Optimizing job parallelism in Apache Flink is key to better performance. It makes sure operators and tasks work together well. This uses your hardware to its fullest.
- Understand Your Data Flow: Look at how data moves and find tasks that can run together. Knowing which tasks depend on each other helps set up parallelism right.
- Resource Allocation: Give each task enough resources to avoid slowdowns. Make sure CPU and memory match the task’s needs.
- Load Balancing: Spread tasks evenly across all resources. Flink’s tools help, but you might need to tweak settings for your needs.
- Task Chain Configuration: Link operators together to cut down on overhead. Flink lets you do this, saving data storage and cutting latency.
By using these tips and following apache flink best practices, your Flink apps will run better. Here’s a quick look at why optimizing job parallelism matters:
Aspect | Before Optimization | After Optimization |
---|---|---|
Resource Utilization | Poor | High |
Processing Speed | Slow | Fast |
Task Execution | Sequential | Concurrent |
System Throughput | Low | Optimal |
In short, focusing on job parallelism optimization and following apache flink best practices boosts your Flink apps’ performance. By fine-tuning parallelism and using resources well, you can make the most of Apache Flink. This leads to top-notch performance for your data tasks.
Effective Use of Checkpoints and Savepoints
Apache Flink checkpoints and savepoints are key for keeping data safe. They help with fault tolerance and stateful stream processing. By tweaking these, companies can boost Apache Flink’s performance and keep data integrity strong.
Configuring Checkpoints
Apache Flink checkpoints help recover data consistently. To set them up right, pick the right interval and timeout. Shorter intervals save data more often, but might slow things down.
- Checkpoint Interval: Set how often to save state. Start with every five minutes.
- Timeout Settings: Set timeouts to handle delays. Make sure they’re shorter than the interval.
Working with Savepoints
Savepoints are vital for updates or restarts. Unlike checkpoints, they’re manually started. They give a snapshot of the state, making updates smooth and safe.
“Effective savepoints management enables Flink developers to rollback or resume processing from a known good state without data loss.”
Using savepoints well can make Apache Flink faster. It cuts downtime during updates and keeps data consistent. Here are some tips:
- Put savepoints in your update pipeline for easy state management.
- Check savepoints often to make sure they work for state recovery.
Configuration Aspect | Recommended Practice |
---|---|
Checkpoint Interval | Every 5 minutes |
Checkpoint Timeout | Less than interval duration |
Savepoints Management | Automate in deployment pipeline |
Savepoints Verification | Regular integrity checks |
Efficient State Management Techniques
Improving state management in Flink is key for top app performance. Pick the right state backend for your app. Options include the default memory backend, RocksDB, or custom solutions based on your needs.
State management in Flink is flexible. Setting state Time-to-Live (TTL) can boost performance. TTL helps get rid of old state, keeping your app efficient and saving resources.
“Effective state management is vital for high-performance Flink applications. By leveraging state backends and TTL configurations, developers can optimize their systems for better scalability and stability.”
Knowing how to optimize Flink for state management is crucial. Here are some tips:
- Choose state backends that fit your app’s needs.
- Use state TTL to manage and clean up state well.
- Keep an eye on your state’s size and performance.
- Use Flink’s metrics to track and improve state performance.
Comparing state backends can give you more insight into their effects:
State Backend | Performance | Scalability | Use Case |
---|---|---|---|
Memory State Backend | High | Limited to memory resources | Low-latency, ephemeral state |
RocksDB State Backend | Moderate | Highly scalable | Persistent, large state |
Custom Implementation | Variable | Depends on the design | Specialized requirements |
Following these Apache Flink optimization strategies keeps your app fast and scalable. Even as state grows and needs increase, these techniques help. Use them to make the most of Apache Flink in your apps.
Leveraging Flink’s Built-in Metrics for Performance Monitoring
Apache Flink has many built-in metrics for monitoring and improving performance. These metrics help find problems and give insights for fixing Apache Flink issues.
Important Metrics to Track
Flink metrics cover a wide range of system and job-specific data. It’s important to watch these metrics:
- JVM Metrics: Keep an eye on heap memory, garbage collection, and GC time for efficient memory use.
- Task Metrics: Track records processed, out-of-order events, and backpressure to check task performance.
- Job Metrics: Look at job duration, downtime, and checkpoint times to see job efficiency.
Using Dashboard Tools
Visualizing Flink metrics helps with quick diagnostics and troubleshooting. Tools like Grafana and Prometheus work with Flink for interactive dashboards. They let you track key metrics easily and show trends and oddities.
Best Practices for Data Processing and Serialization
Optimizing data processing in Flink is key for high performance and scalability. Knowing how to serialize data well is also important. Bad serialization can cause delays and lower speeds.
- Use windowing to manage data streams well.
- Take advantage of Flink’s operators like joins and filters.
- Make sure your data pipeline works for both batch and stream processing.
For serialization best practices, follow these tips:
- Pick a serialization framework that fits your app, like Avro or Protobuf.
- Adjust serialization settings to make data smaller and faster to load.
- Test different frameworks to find the best one for your needs.
Here’s a quick look at popular serialization frameworks:
Framework | Strengths | Weaknesses |
---|---|---|
Avro | Schema evolution, compact format | Slower serialization/deserialization speed |
Protobuf | High performance, language agnostic | Complex schema definition |
Kryo | Fast serialization, supports custom serializers | Limited support for schema evolution |
In summary, using best practices for data processing in Flink and following serialization tips can greatly improve your app’s performance. Remember, the right choices in these areas can make a big difference in speed and efficiency.
Apache Flink Performance Optimization Strategies
To make Apache Flink apps run better, you need to know how to tweak network buffers and set up the RocksDB backend. These steps help keep your app fast and efficient. They also make managing data easier.
Tuning Network Buffers
Adjusting network buffers is key to better performance in Apache Flink. Changing these settings can greatly affect how fast and smooth your app runs. First, find the right buffer size by balancing memory use and speed.
Keep an eye on how much data is flowing through these buffers. This helps avoid slowdowns in your app.
Configuring RocksDB Backend
Setting up the RocksDB backend is crucial for managing data well. The right RocksDB settings can make your app with lots of data run much better. You need to fine-tune memory use, compaction, and write buffers based on your app’s needs.
Try out different settings to see what works best for your app. This way, you can get the most out of your data management.
Conclusion
Optimizing Apache Flink’s performance is a big job. It needs a mix of understanding, setting up a scalable cluster, and fine-tuning. This guide shows how these steps are key to making your apps efficient and strong.
Monitoring your app’s performance is crucial. Use Flink’s tools to get real-time data. This helps you make quick changes. Managing checkpoints and state well also boosts your app’s reliability and speed.
Pay close attention to how your jobs run, task manager settings, and how you handle data. These steps are important for your Flink apps to do well. By following these tips, your apps will not only meet but go beyond your expectations, handling data efficiently.
FAQ
What are some common performance bottlenecks in Apache Flink?
Apache Flink often faces issues like bad parallelism and state management. Also, resource allocation, network problems, serialization, and backpressure can slow it down.
How can I identify symptoms of poor Apache Flink performance?
Look for signs like high latency and out-of-memory errors. Also, watch for high CPU use, backpressure, and uneven task distribution. These signs help you know where to improve.
What are the best practices for setting up a scalable Flink cluster?
For a scalable Flink cluster, pick the right hardware and consider cloud options. Make sure your cluster is stable and scalable. These steps can really help your Flink app run better.
How should I configure memory for better Task Manager performance in Flink?
Set the task heap, managed memory, and direct memory limits right. Adjust these based on your workload. This is key for making your Task Manager run smoothly.
Why is job parallelism important in Flink, and how can it be optimized?
Job parallelism lets tasks run at the same time, using your hardware better. To improve it, use `setParallelism` and make sure tasks are spread evenly.
What is the role of checkpoints and savepoints in Flink?
Checkpoints and savepoints help Flink recover from failures and manage state. They make your stream processing more reliable. Setting them up right can boost your Flink’s performance.
What techniques can be used for efficient state management in Flink?
For better state management, choose the right backend like RocksDB. Use state TTL to avoid old state. Also, make sure state access is efficient to reduce overhead.
Which Flink metrics are important to track for performance monitoring?
Track task time, throughput, checkpoint duration, buffer use, and backpressure. Dashboard tools can help you monitor these and improve your Flink.
What are some best practices for data processing and serialization in Flink?
Use fast serialization like Kryo or Avro. Avoid extra data transformations. Make your data processing fast and efficient.
How can network buffers impact Flink performance, and how should they be tuned?
Network buffers control data flow. Tune them to balance latency and throughput. This is crucial for Flink’s performance.
What are the benefits of configuring the RocksDB backend in Flink?
RocksDB backend offers efficient state storage and retrieval. Tuning its settings can greatly improve your Flink’s performance.
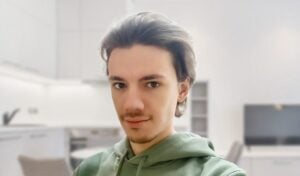
- Understanding Apache Flink Performance Challenges
- Setting Up a Scalable Flink Cluster
- Tuning Task Manager Configuration
- Optimizing Job Parallelism
- Effective Use of Checkpoints and Savepoints
- Efficient State Management Techniques
- Leveraging Flink’s Built-in Metrics for Performance Monitoring
- Best Practices for Data Processing and Serialization
- Apache Flink Performance Optimization Strategies
- Conclusion
- FAQ
Explore more topics
Talk to Our Talent Expert
